every()
用于判断数组所有元素是否都符合函数返回的条件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> </head> <body> <script> var array=[86,3,2,52,1];
var result=array.every((i)=>{ return i>2; }); console.log(array); console.log(result); </script> </body> </html>
|
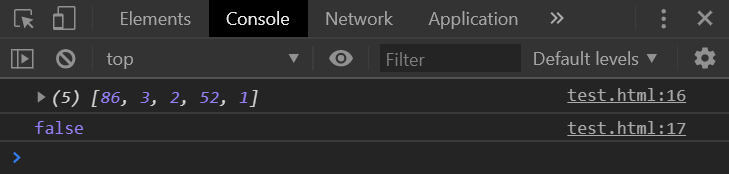
some()
用于判断数组所有元素中是否有一条满足函数返回的条件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> </head> <body> <script> var array=[86,3,2,52,1];
var result=array.some((i)=>{ return i>2; }); console.log(array); console.log(result); </script> </body> </html>
|
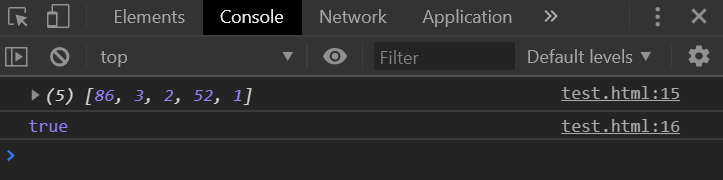
find()
用于查找数组中第一个满足条件的元素,并返回
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>js find</title> </head> <body> <script type="text/javascript"> var array=[3,2,52,1]; var result=array.find((i)=>{ return i>2; }); console.log(array); console.log(result); </script> </body> </html>
|
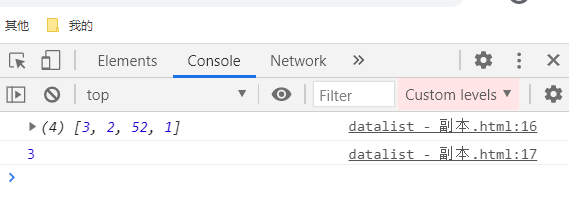
filter()
用于过滤数组所有满足条件的元素,并返回
和every()有些像,但那是返回 ture或false,而filter()是返回符合条件的数组
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> </head> <body> <script> var array=[86,3,2,52,1];
var result=array.filter((i)=>{ return i>2; }); console.log(array); console.log(result); </script> </body> </html>
|
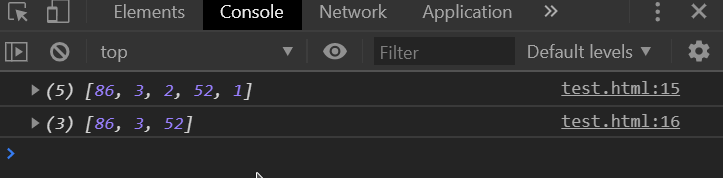
map()
处理数组的每个元素,并返回处理后的数组。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> </head> <body> <script> var array=[86,3,2,52,1];
var result=array.map((i)=>{ return i*2; }); console.log(array); console.log(result); </script> </body> </html>
|
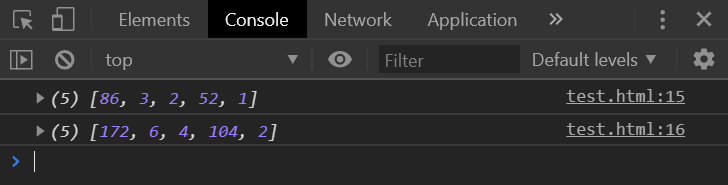
reduce()
用于累加计算,返回结果
接收两个参数:function和params。function有有四个参数total, now, index, array。total是前值和后值得累加值,now是当前值,index索引,array数组自身。后两个非必传。params非必传,传递给函数的初始值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> </head> <body> <script> var array=[86,3,2,52,1,55];
var result=array.reduce((total, now, index, array)=>{ console.log(total, now, index, array); return total + now; }); console.log(result); </script> </body> </html>
|
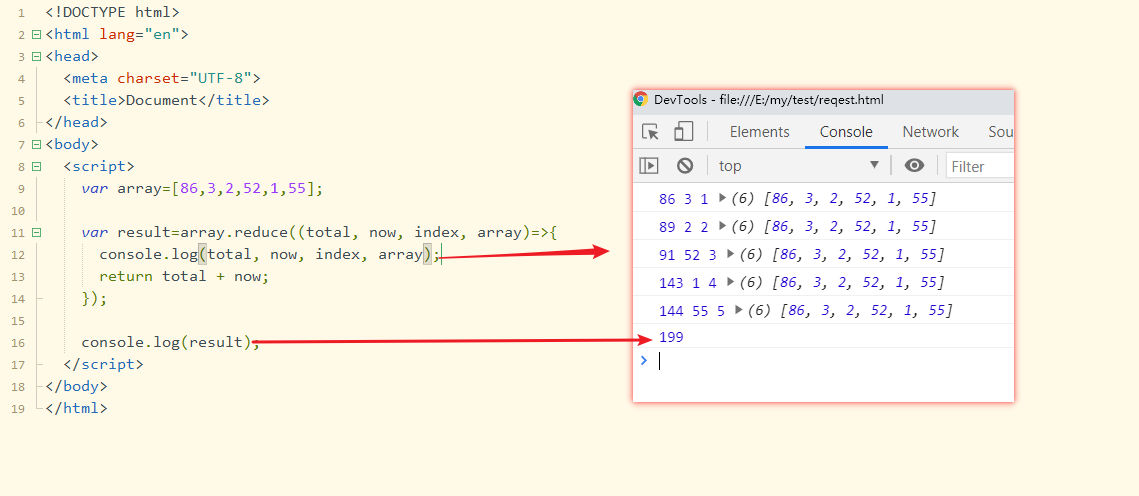